Problem Statement:
How to render out everything in your world so that when when a character is behind an object then parts of that character are not visible and when you are in front of an object then you are completely visible?
In 3D engines it is sort of easy, the rendering is done with the help of a ZBuffer. And in Libgdx actually everything is rendered with a 3D engine, although you will not immediately notice.
In 2D Engines you need to start laying out how your scene should be drawn with the help of some data structure. Mostly your scene contains a background, a part of that background that is more of a backdrop of your character and some parts of the scene are only in the foreground. The tricky bits are the objects where the character can move in front of or move behind it.
The best way to display my world is to use the good old ‘Painters algorithm’ that basically says. Start with the stuff that’s farthest away, they layer each other part that is closer to your eye. The eye in your game is the camera.
The best way to display my world is to use the good old ‘Painters algorithm’ that basically says. Start with the stuff that’s farthest away, they layer each other part that is closer to your eye. The eye in your game is the camera.
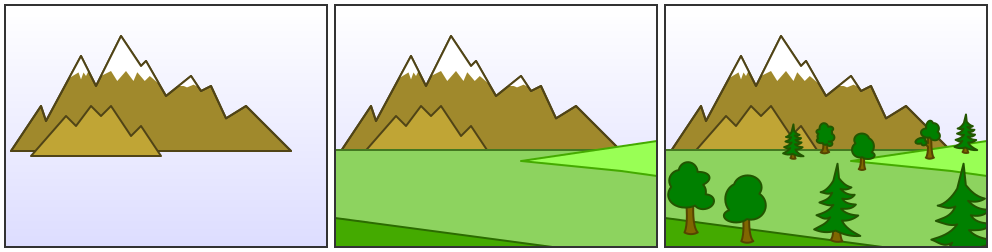
One way to identify the order of painting is with some kind of number. You could say that, the higher the number , the farther it is away from the eye (camera).
{
name: "painting"
usedir: "DIR_BACK"
zsort: 109
animations: [
{
name: "painting"
frames: [
"painting"
]
}
]
hotspot: ((-30,-22),(31,22))
pos: (253,178)
usepos: (0,-86)
}
The Wimpy tool’s output has a field zsort that allows me to identify a sort of ordering number and also a usedir that I can use to indicate it is part of the backdrop. I think that the property is actually useable for the direction in which the character should face when it is (for example) looking at the painting.
The same I could do with objects that are always show in front of anything else. Those objects can have a usedir with “DIR_FRONT”. For example the next picture shows that the character always is behind of the chandelier, but always in front of the mirror.
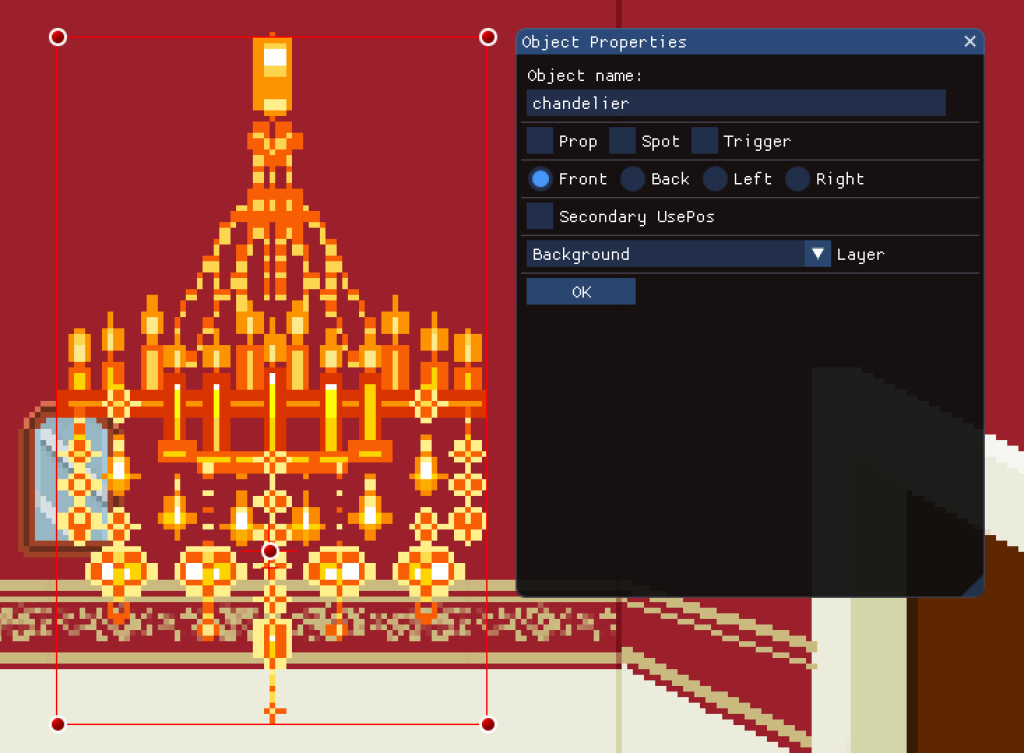
But those objects that are present IN the room. How should these be handled? You could restrict those objects to have a rectangular form so that it is easy to check if the character overlaps on the front part or overlaps on the back part.
But that is restrictive when you have some interest perspective angles on certain items.

But luckily there is a way to do it…. Let’s show the strategy with two pictures :

Remember the MINIMUM Y-Position for all the intersected points with ray and the polygon.
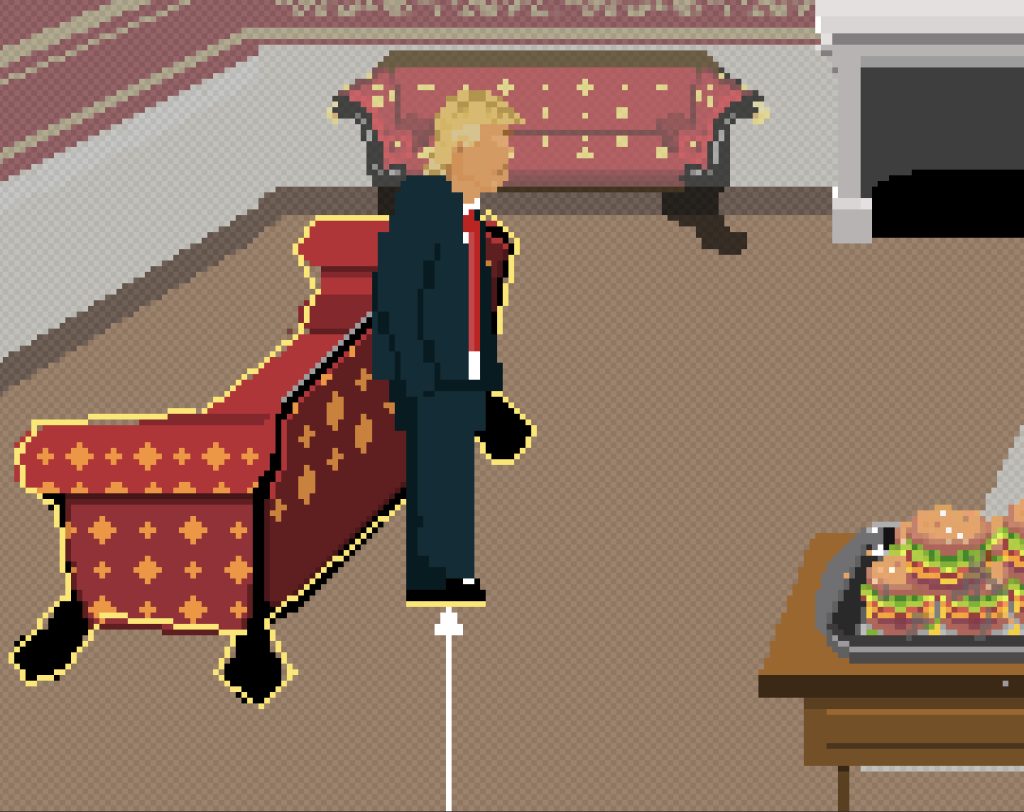
Remember the MINIMUM Y-Position of the characters ‘base’ (can be derived from the bounding box)
If the characters Y-Pos is lower than the objects Y-Pos, than the character is in front of the object, otherwise it the character is behind it.
This works really well and of course it is not perfect as the character could stand between the two legs in front of the big sofa. But you could either restrict movement there or make the polygon closed and more convex.
Finally a video where Zsorting and the surrounding ‘hidden’ world that makes it happen.. is shown: